Linker errors, such as “undefined reference” or “unresolved external symbol,” can make it difficult for C++ developers to move ahead. This type of error occurs especially if the code seems to be compiled, and linking fails. It also occurs when the linker cannot find the definition of a declared function, variable, or object. The common cause lies in the function definitions being missing, incorrect signatures, missing object files, or libraries not being linked. Here is an article where we will discuss the errors, their common causes, methods for their effective fixing, and some example cases.
Table of Contents:
Linker Errors in C++
Linker errors such as “undefined reference” or “unresolved external symbol” occur, especially if the code seems to be compiled and linking fails. It also occurs when the linker cannot find the definition of a declared function, variable, or object. The common causes lie in the function definitions being missing, incorrect signatures, missing object files, or libraries not being linked.
1. What is an Undefined Reference Error in C++?
An undefined reference error comes up any time the linker cannot find the definition of a function or variable that has been declared and used in the program. Thus, unlike a compilation error, which stops the program from being compiled, an undefined reference indicates that the linking phase could not find an implementation of a product that was recognized by the compiler inside the source file.
Example:
Output:
Explanation: The code shows how a function, myFunction(), is declared but is not defined, which leads to an undefined reference error during the linking phase because the linker doesn’t find its implementation.
2. What is an Unresolved External Symbol Error in C++?
An unresolved external symbol error is the equivalent of an MSVC undefined reference error. It happens whenever an object file or library is missing in the linking phase, which results in the lack of function or variable definitions.
Example:
Output:

Explanation: The code shows how a function myFunction() is declared but is not defined, which leads to an unresolved external symbol error during the linking phase because the linker cannot find its implementation.
Causes of Undefined Reference / Unresolved External Symbol Errors in C++
- When you declare a function, but no linking files contain a definition for that function, you will have an undefined reference to that function.
- When the declaration and definition of a function are not matched, it generates a linker error.
- If an object file containing a function definition is not linked, the linker cannot resolve the reference.
- If you are using a static library and you may not have linked it correctly, it will result in an unresolved external symbol.
- Template and inline functions have to be defined in the header file to avoid linking trouble.
- If the function is inside a namespace but called without specifying it clearly, the linker cannot find it.
- A pure virtual function must be implemented in a derived class, or the linker will generate an error.
Fixes for Undefined Reference / Unresolved External Errors in C++
- Missing Function Definition: Make sure that every function that is declared is defined.
- Incorrect Function Signature: Ensure that the function declaration and definition match.
- Undefined Class Member Function: Provide a definition for ClassName::method().
- Static Library Not Linked: Make sure that .a (GCC) or .lib (MSVC) file is linked before compilation.
- Virtual Function Not Defined: Provide an implementation for all pure virtual functions of derived classes.
- Namespace Issues: Use Namespace::Function() or use a directive to access functions.
- Inline and Template Issues: You have to define the inline and template functions in header files.
Common Cases and Fixes for Undefined Reference / Unresolved External Symbol Errors in C++
1. Missing Function Definition
A missing function definition is an error that occurs when a function is declared but not defined anywhere in the linked files, which causes the linker to generate an undefined reference error.
Example:
Output with Error:

Explanation: The above code declares myFunction() but does not provide a definition for the same, which causes the linker to not find its implementation and results in an undefined reference error during the linking phase.
How to Fix it: Provide the function definition.
Example:
Output:

Explanation: The code ensures that the linker can easily find the function’s implementation, resolving the error.
2. Incorrect Function Signature
An incorrect function signature is an error that occurs when the function declaration and definition do not match exactly, which makes the linker to fail in resolving the function call.
Example:
Output with error:
Explanation: The above code produces an undefined reference error because the function declaration (void myFunction(int x);) expects an int parameter, but the definition (void myFunction()) does not take any parameters, due to which the linker cannot match the function call myFunction(10) with the existing definition.
How to Fix it: Ensure the function definition matches the declaration.
Example:
Output:

Explanation: The code shows how the function definition matches the declaration, which allows the linker to resolve the function call without any error.
3. Inline Function Issues
These are the undefined reference errors that occur when inline functions are declared in a unit but not defined in the same unit, as it prevents the linker from locating a definition for the inline functions.
Example:
Output with Error:
Explanation: The code shows that the inline function myFunction() is declared but not defined, which causes the code to produce an undefined reference error.
How to Fix it: Define the inline function in the same file or header.
Example:
Output:

Explanation: The code shows how the compiler avoids the linking error by substituting the inline definition calls to myFunction().
4. Template Function Issue
A template function should be defined in the same translation unit or inside a header file, as the compiler must generate its definition for each type used. If the definition is not present, the linker will generate an undefined reference error.
Example:
Output with Error:

Explanation: The given code produces an undefined reference error because the template function add(T a, T b) is declared but not defined, which means that the full definition should be located in the same translation unit.
How to Fix it: Define the template function in the same file or a header.
Example:
Output:

Explanation: The above code defines and uses a template function correctly. The template function add(T a, T b) has both a declaration and definition in the same file, which allows the compiler to generate the appropriate instantiation of the function when it is called with add(3, 4).
5. Virtual Function Not Defined in Derived Class
A pure virtual function in a base class must be implemented in any derived class. If it is missing, the linker will generate an undefined reference error, and the class remains abstract, preventing object instantiation.
Example:
Output with Error:
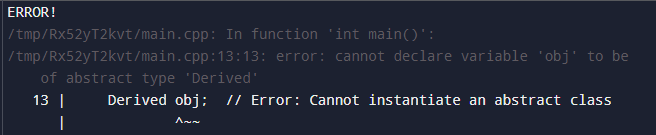
Explanation: The code results in a linker error because the pure virtual function display() is declared in the Base class but is not implemented in the Derived class. Since Derived does not provide a definition for display(), it remains an abstract class and cannot be instantiated.
How to Fix It: Implement the Pure Virtual Function in Derived Class
Example:
Output:

Explanation: The code correctly implements a pure virtual function in the derived class, allowing successful compilation and execution.
6. Namespace and Scope Issues
A function or variable declared inside a namespace must be referenced using the namespace qualifier unless using a directive. Calling the function without proper qualification would restrict the linker from finding its definition, which will lead to an undefined reference error.
Example:
Output with Error:
Explanation: The above code results in a linker error because certain functionalities are defined inside the namespace MyNamespace, but they are called without specifying the namespace qualification in main(). The compiler thus does not find myFunction() in the global scope, which will lead to an undefined error.
How to Fix It: Use the Namespace Qualifier or Directive
Example:
Output:

Explanation: The code calls myFunction() with the correct namespace qualifier so the compiler can find and link the function without any error.
Conclusion
Undefined references and unresolved external symbol errors will occur when the linker cannot find a definition for a declared function or variable. Mostly, the errors arise from missing functions, how the function is typed, missing object files, and poorly linked libraries. Fixes for such errors require that all functions are defined properly, the function signatures and declarations are matched, and various necessary files are linked, as well as the handling of templates, inline functions, and namespaces.
What are undefined reference/unresolved external symbol errors in C++ – FAQs
Q1. What creates an undefined reference error in C++?
An undefined reference error occurs when a function or variable is declared but not defined during the linking phase.
Q2. How would I fix an undefined reference error?
Make sure every declared function and variable has proper definitions, corresponds to mismatches, and links with needed libraries or object files.
Q3. What’s the difference between an undefined reference and an unresolved external symbol?
They are the same fault type, but “undefined references” is a common name in GCC while “unresolved external symbol” is the equivalent term in MSVC.
Q4. Can undefined reference errors occur when a function signature is wrong?
Yes, if a function declaration does not perfectly match its definition, then the linker cannot resolve it, resulting in the error.
Q5. Why do inline and template functions generate linker errors?
Inline and template functions need to be completely defined in the header file because they are instantiated at compile time. If only declared but not defined in the same translation unit, the linker cannot resolve them, resulting in an error.