While working with Java, comparing two strings is the most common task, when handling user input, database queries, or implementing business logic. Java provides us with different methods to compare two strings in Java.
In this blog, we are going to learn how we can compare two strings in Java using the String.equalsIgnoreCase() method.
Table of Contents:
What is the equalsIgnoreCase() Method in Java?
The equalsIgnoreCase() method in Java compares two strings irrespective of their case(either uppercase or lowercase). It returns true if the value of both strings is the same. This method is used in situations where case sensitivity is not the concern, such as in user input validation or search operations.
Syntax:
boolean result = string1.equalsIgnoreCase(string2);
Parameters:
- string1 is the first string to compare.
- string2 is the second string to compare with string1.
- result is a boolean value that will be true or false.
Return value: The return type of this function is a boolean value(either true or false).
Example 1: Comparing two strings in Java
Let’s take a look at the below example that compares two strings irrespective of their case(either uppercase or lowercase).
Code:
Output:

Explanation: In the above program, str1 and str2 have the same value, the first one is in uppercase, and the second string is in lowercase, The equalsIgnoreCase() method returns true for str1.equalsIgnoreCase(str2) because it ignores case differences. However, str1 and str3 do not have the same value, so it returns false.
Example 2: Comparing User Input Strings in Java
Let’s take a look at the below example that takes two strings as input and checks whether these two strings are equal or not.
Code:
Output:
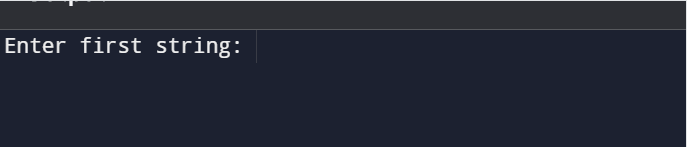
Explanation: In the above code, we have taken two input strings from the user, and checked whether these are the same or not, if both strings are the same, it prints the output “The strings are equal (ignoring case)”, otherwise prints the output “The strings are not equal.”
Conclusion
So far in this blog, we have learned how we can compare two strings irrespective of their case( either lowercase or uppercase). The equalsIgnoreCase() method helps you to perform case-insensitive string comparisons, it is helpful in scenarios like user authentication and form validations.
If you want to be an expert in Java Programming language, you may refer to our Java Course.
Some Other Methods to Compare Strings in Java
- Using == Operator
- Using Objects.equals() Method
- Using String.compareTo() Method
- Using compareToIgnoreCase() Method
- Using startsWith() Method
- Using endsWith() Method
- Using User-Defined Function