Forms on the webpage allow the user to send their data to the server. Sometimes you want to stop the data from submission to prevent accidental submission, check the user information, and handle the user input in a different way. Let’s discuss some of the methods to stop form submission using JavaScript in this blog.
Table of Contents:
Method to Stop Form Submission Using JavaScript
You can use the methods such as event.preventDefault(), stop form submission on button click, and return false method. Let’s discuss these methods below.
Method 1: Using event.preventDefault()
You can use this method to prevent the default action of an event from occurring. In the case of form, event.preventDefault() function stops the form from being sent to the server side.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Prevent Form on Button Click</title>
</head>
<body>
<form id="myForm">
<button id="submitButton" type="submit">Submit</button>
</form>
<script>
const submitButton = document.getElementById("submitButton");
submitButton.addEventListener("click", function(event) {
event.preventDefault();
alert("Submission stopped on button click!");
});
</script>
</body>
</html>
Output:
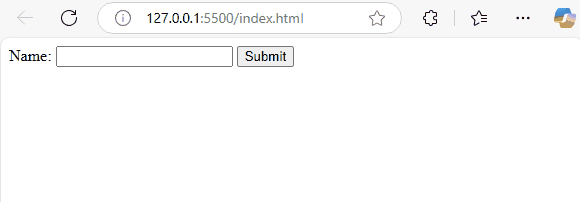
Explanation: When you try to submit the form with the text input for your name and the submit button, the JavaScript code stops the form from being submitted to the server. The JavaScript code contains a document.getElementById(“myForm”) to find the form and event.preventDefault() stops the default action of the form (which normally sends data to the server). The alert message pops up finally.
Method 2: Stopping Form Submission on Button Click
This method targets the button rather than the form. Here the targetted event is click not submit event. This approach works well when you want to stop the user from submitting the form in the case of requiring additional information before submitting the form.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Prevent Form on Button Click</title>
</head>
<body>
<form id="myForm">
<button id="submitButton" type="submit">Submit</button>
</form>
<script>
const submitButton = document.getElementById("submitButton");
submitButton.addEventListener("click", function(event) {
event.preventDefault();
alert("Submission stopped on button click!");
});
</script>
</body>
</html>
Output:
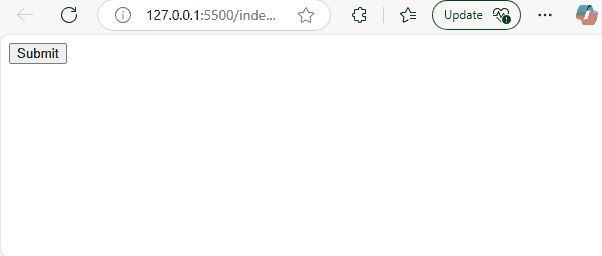
Explanation: You can use this code to stop the form from submission. When the user clicks the button, the JavaScipt function runs and stops the form from the submission. Function event.preventDefault() is used for this purpose. And finally, the user gets the alert message.
Method 3: Using the Return False Method
You can use this method to prevent the submission of the form. This method is considered to be the older technique compared to other methods. This method returns false when it stops the submission.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Return False Example</title>
</head>
<body>
<form onsubmit="return preventSubmission()">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<button type="submit">Submit</button>
</form>
<script>
function preventSubmission() {
alert("Form submission stopped!");
return false; // Prevents the form from being submitted
}
</script>
</body>
</html>
Output:
Explanation: You can use the form onsubmit attribute in the HTML to call the JavaScript function preventSubmission(). This match is defined in the script tag. You will get an alert message stating form submission stopped. This function then returns false, preventing the form from getting submitted.
Conclusion
The above-discussed methods are the most effective approach to stop the form from submission. You can use the JavaScript function like event.preventDefault() and return false and stop the form on button click methods for this purpose. Therefore you can provide flexibility for the users by preventing accidental submissions, this makes the webpage more user-friendly.
How to Stop Form Submission in JavaScript – FAQs
1. Why would I want to stop form submission?
You can stop the form submission to ensure the user input is valid, prevent accidental submission, and handle the user’s input in a customized way.
2. How can I stop form submission using JavaScript?
You can use the event.preventDefault(), stopping the submission on button click, and using the return false method.
3. What is event.preventDefault() and how does it work?
You can prevent the default action like form submission using event.preventDefault(). It works by stopping the form sent to the server.
4. How do I stop form submission on a button click?
You can use the event.preventDefault() within the event listener to stop the form submission.
5. How do I stop form submission on a button click?
You can use the return false function in the form onsubmit event to stop the user from form submission.