File handling in C++ is crucial for storing and retrieving data. It enables programs to read from and write to files, ensuring persistent data storage. This functionality is widely used in applications like databases, text editors, and games. Nearly every programming language includes a ‘file handling’ method for data storage, and this blog will help you understand C++ file handling. If you’re new to C++, this blog will guide you through understanding how C++ manages files, an essential skill for effective data storage and recovery in programming.
Table of Contents
Watch the video below to understand C programming in detail:
What is File Handling in C++?
File handling in C++ refers to the process of working with files, reading from them, writing to them, and manipulating their contents. It involves using streams such as input streams (for reading) and output streams (for writing).
In the C++ programming language, you can perform file handling operations by including the “<fstream>” header, which provides classes like “ifstream” (for input operations), “ofstream” (for output operations), and “fstream” (for both input and output operations). These classes allow you to open files, read data from them, write data to them, close files, and perform various operations like seeking a specific position within a file or checking the end-of-file condition.
Do you want to jumpstart your career in computer programming? Enroll in our C Programming Course and gain the skills to succeed!
What are the Primary Classes for File Handling in C++?
In C++, there are three primary classes used for file handling:
- std::ifstream – Input file stream class used to read from files.
- std::ofstream – Output file stream class used to write to files.
- std::fstream – File stream class that supports simultaneous input and output operations on files.
These classes are part of the C++ Standard Library and provide functionalities to perform various file operations such as reading from files, writing to files, opening, closing, and manipulating file contents.
Before stepping into file operations in C++, it is essential to know about the Naming Conventions of a file. Choosing a meaningful and descriptive name for a file is crucial for effective file handling. The naming conventions should be short, meaningful, and concise. One should avoid special characters, spaces, and reserved keywords while naming a file. Some examples of naming conventions are given below:
Good Naming :
- sales_report_2022.xlsx
- user_preference.json
- main_script.py
Avoid:
- file123.txt (non-descriptive)
- important file.doc (contains space)
- student%file.txt (contain special character)
File Operations in C++
File operations in C++ involve reading from and writing to files. They are crucial for data storage, retrieval, and manipulation; enabling programs to interact with external files, and facilitating data persistence and sharing across applications.
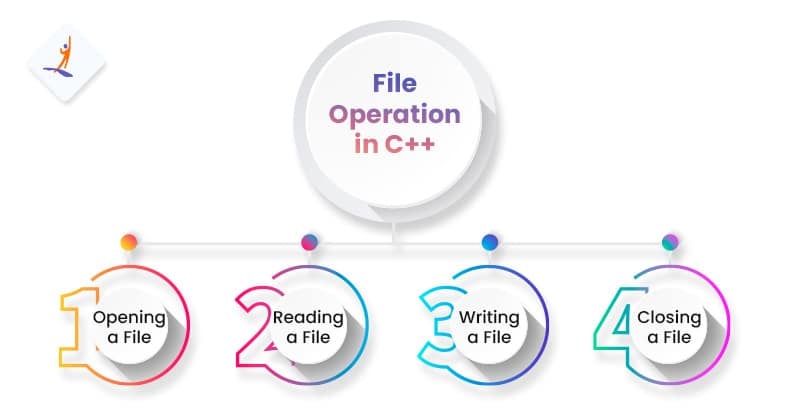
Opening a File in C++
In C++, opening a file refers to the process of establishing a connection between the program and a file on the disk. This connection allows the program to perform various operations on the file, such as reading or writing.
Syntax:
open( FileName , Mode );
FileName: Denotes the name of the file
Mode: Denotes the mode of the file in which you want to open it
The below table shows the different types of modes to open a file:
Modes | Description |
iso::in | Opening file in reading mode |
iso::out | Opening file in write mode |
iso::app | Opening file in append mode |
iso::ate | Opening file in append mode, but read and write will be done at the end of the file |
iso::binary | Opening file in binary mode |
iso::trunc | Opening file in truncate mode |
iso::nocreate | Opening file only if it exists |
iso::noreplace | Opening file only if it doesn’t exist |
Code:
#include <iostream>
#include <fstream>
int main() {
// Declare an object of the fstream class
std::fstream file;
// Open a file named "example.txt" in output mode
file.open("example.txt", std::ios::out);
// Check if the file is successfully opened
if (!file.is_open()) {
std::cerr << "Error opening the file!" << std::endl;
return 1; // Return an error code
}
// Write some data to the file
file << "Hello, File I/O in C++!" << std::endl;
// Close the file when done
file.close();
std::cout << "File successfully written." << std::endl;
return 0;
}
Output:
File successfully written.
Check out the top C++ Interview Questions to ace your next interview!
Reading a File in C++
Reading from a file involves using the >> operator (or other appropriate read functions provided by the file stream object) to extract data from the opened file into variables or buffers.
Syntax:
fileStream >> variable; // Reading data from the file
Code:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream infile("example.txt"); // Opens the file for reading
if (infile.is_open()) {
std::string line;
while (std::getline(infile, line)) {
std::cout << line << "\n"; // Output each line from the file
}
infile.close(); // Close the file when done reading
} else {
std::cout << "Unable to open the file!\n";
}
return 0;
}
Writing a File in C++
Writing to a file involves using the << operator (or other appropriate write functions provided by the file stream object) to write data into the opened file.
Syntax:
fileStream << "Data to write to the file"; // Writing data to the file
Code:
#include <iostream>
#include <fstream>
int main() {
std::ofstream outfile("example.txt"); // Creates/Opens a file called "example.txt" for writing
if (outfile.is_open()) {
outfile << "Hello, file! This is some text.\n";
outfile << "Another line in the file.\n";
outfile.close(); // Close the file when done writing
std::cout << "Data has been written to the file.\n";
} else {
std::cout << "Unable to open the file!\n";
}
return 0;
}
Output:
Data has been written to the file.
Know the concepts of OOPs in C++ with our comprehensive blog!
Closing a File in C++
Closing a file involves using the “close()” function on the file stream object to terminate the connection between the program and the file. This operation is important to ensure that all data is properly written or read and to release system resources associated with the file.
Syntax:
fileStream.close(); // Close the file
Code:
#include <iostream>
#include <fstream>
int main() {
std::ofstream fileStream("example.txt");
if (fileStream.is_open()) {
// Perform operations with the file (writing, etc.)
fileStream.close(); // Close the file
std::cout << "File closed successfully.\n";
} else {
std::cout << "Unable to open the file!\n";
}
return 0;
}
Output:
File closed successfully.
Interested in building projects? Here are some of the Top 20 C++ Project Ideas!
File Position Pointers
File position pointers, also known as file pointers or file position indicators, are used in file I/O operations to keep track of the current position in a file. They represent the location within the file where the next read or write operation will occur. In C++, file position pointers are associated with file stream objects (std::fstream, std::ifstream, std::ofstream).
The two primary file position pointers are as follows:
get pointer (“get pointer” for input streams):
- It represents the current position for the next character to be read from the file.
- It is controlled by functions such as “tellg()” (to get the current position) and “seekg()” (to set the position).
put pointer (“put pointer” for output streams):
- It represents the current position for the next character to be written to the file.
- It is controlled by functions such as “tellp()” (to get the current position) and “seekp()” (to set the position).
Example of using file position pointers:
#include <iostream>
#include <stream>
int main() {
std::fstream file("example.txt", std::ios::in | std::ios::out);
if (file.is_open()) {
// Get the current get pointer position
std::streampos getPosition = file.tellg();
std::cout << "Current get pointer position: " << getPosition << "\n";
// Move the get pointer to a specific position (e.g., the beginning of the file)
file.seekg(0, std::ios::beg);
// Get the current put pointer position
std::streampos putPosition = file.tellp();
std::cout << "Current put pointer position: " << putPosition << "\n";
// Move the put pointer to a specific position (e.g., the end of the file)
file.seekp(0, std::ios::end);
file.close();
} else {
std::cout << "Unable to open the file!\n";
}
return 0;
}
Output:
Current get pointer position: 0
Current put pointer position: 0
In this example, “tellg()” and “tellp()” are used to retrieve the current positions of the get and put pointers, respectively. The “seekg()” and “seekp()” functions are then used to move the pointers to different positions within the file. These operations are useful for navigating and manipulating the content of a file during input and output operations.
Checking State Flags
In C++, the std::ios class provides various state flags that help in understanding the state of an input/output stream. These flags can be accessed and checked using member functions to understand if certain conditions occurred during I/O operations.
Here are some common flags and their usage:
fail(): Checks if an operation failed (equivalent to “bad() | fail()”).
eof(): Checks if the end-of-file has been reached.
bad(): Checks if a non-recoverable error has occurred.
good(): Checks if no error flags are set (opposite of “fail()”).
Concluding Thoughts
File handling in C++ remains fundamental for data persistence and manipulation. With evolving technologies, its importance extends to database management systems, large-scale data processing, and cross-platform applications. As data continues to be a cornerstone, mastering file handling ensures robust data management, facilitating future innovations in software development.