Introduction:
A loop in programming is a control statement that allows you to execute a block of code repeatedly, either when a condition needs to be met or for a particular condition needs to be met. Now suppose you want to print a sequence of numbers, suppose 1-100, You would have to write the print statement 100 times. But in the case of using a loop for the same task, you would be doing the same thing by writing just a few lines of code. In this blog, we are going to learn about the two most important loops and also compare them to understand which loop to use in which condition.
Table of Contents:
What is Python for loop?
A for loop in Python is a control flow statement that is used to iterate over a sequence like a list, tuple, range, dictionary, etc. It executes a block of code a specific number of times or for each element in the sequence, therefore making it more useful for performing repetitive tasks such as processing data, printing values, or even performing calculations.
Flowchart of Python for loop:
Python for loop Syntax:
for iterator in sequence:
#Code block to execute
Iterator: gives the current element
Sequence: The iterable (e.g. list, range) to iterate through.
Example of Python for loop:
colors = ['red', 'yellow', 'blue'] for color in colors: print(color)
Explanation:
The code above is an example of a for loop where there is a list created with the variable name colors. Now we are iterating our for loop over the list with the iterator name as color and printing all the elements present in the list.
Output:
red yellow blue
What is Python while loop?
A while loop in Python is another control flow statement that is used when you want to repeatedly execute a code as long as a specified condition is true. It is used when the number of iterations is not determined and it depends on a condition.
Start Your Journey to Python Excellence
Explore Python Like Never Before
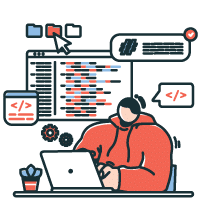
Flowchart of Python while loop:
Python while loop Syntax
while condition:
#Code to be executed as long as the condition is true
while: indicates the beginning of the loop
condition: The expression that is evaluated at the beginning of each iteration.
Example of Python while loop
count = 0 while count < 5: print(count) count += 1
Explanation:
The block of code above executes as long as the conditions are True. Therefore, according to the block of code, the condition is the code will execute as long as the value of count variable is less than 5.
Output:
0 1 2 3 4
Difference Between For Loop and While Loop in Python
For Loop | While Loop |
It uses for keyword. | It uses while keyword. |
It is used to iterate over a sequence. | It is used for the execution of a block of code as long as the condition is met. |
It is used when the number of iterations are known beforehand | It is used when the number of iterations are unknown or dynamic. |
Syntax: for item in sequence: #code | Syntax: while condition: #block of code |
It stops when it completes the traversal of the sequence or range. | It stops when the condition becomes false. |
When to use For Loop and While Loop in Python?
A for loop is used when the number of iterations is known or when you are required to iterate over a sequence like a list, tuple, string, or range. It is mostly used for tasks like traversing over a collection, performing in particular operation over each element, or running a block of code a fix number of times. For example, if you want to print all the names of the students present from an attendance sheet:
students = ["Nisha", "Bobby", "Disha", "Isha"] # Using a for loop to print each student's name for student in students: print(f"Present: {student}")
#Output:
Present: Nisha Present: Bobby Present: Disha Present: Isha
Its concise syntax makes it ideal for scenarios where the iteration is predictable or straightforward.
On the other hand, a while loop can be used when the number of iterations is not known beforehand and also depends on the condition that might change from time to time. It is mostly used for tasks where you want to take user input or when you want to perform certain operations based on some conditions. For example, you can use a while loop when you repeatedly want to take user input or repeatedly want to prompt the user to provide a valid input. For example:
number_to_enter = 0 # Initialize with an invalid value while number_to_enter <= 0: # Repeat until the user enters a valid number number_to_enter = int(input("Enter a number greater than 0: ")) if number <= 0: print("Invalid input. Please try again.") print(f"Thank you! You entered: {number_to_enter}")
Output:
Enter a number greater than 0: -3 Invalid input. Please try again. Enter a number greater than 0: 0 Invalid input. Please try again. Enter a number greater than 0: 5 Thank you! You entered: 5
What happens in the Absence of Condition
In a for-loop, when a condition is absent, the loop will not execute at all, but it will not show any error. For example, if you give any empty list or any empty range, the loop block of code will be skipped and the other parts of the program will be executed.
For example:
for i in range(0, 0): # No numbers in the range print("This will not run.")
In the above example, we have provided an empty range, and thus the loop will be skipped entirely without raising any error.
In the case of the while loop, removing the condition will cause a syntax error because the loop needs a condition to evaluate if it should continue executing. Without a condition, Python cannot decide when to stop the loop, thus causing a failure in the program’s syntax. Now if a condition like True is used, however, then the loop will execute indefinitely, potentially leading to an infinite loop. It is important to make sure that the while loop has a valid condition that would be evaluated as False to prevent any such scenarios.
For example:
while: # SyntaxError due to missing condition print("This will cause an error.")
Get 100% Hike!
Master Most in Demand Skills Now!
Conclusion:
In conclusion, for and while are both important iteration functions in Python. While a for loop is pretty useful for more predictable situations, such as knowing how many times you’ll have to do something or iterating over a sequence, like a list or a range, a while is reserved for situations where the number of iterations is not determined until runtime and depends on some condition or variable. Knowing when to use each type of loop ensures efficient, readable, and error-free code. If you want to explore more in this domain, you can enroll in Intellipaat’s Python Course and give your career the right choice.