Detecting a click outside an HTML element is a common task in web development. Whether you are working with the dropdown menus or modals (a UI element that temporarily displays over the website), you need to close or hide the element when the user clicks outside of the element.
In this blog, you will understand the method to detect outside clicks in JavaScript. Also, you know various best practices to ensure a smooth user experience.
Table of Contents:
Understanding Event Bubbling and Capturing
Before learning about the method to detect clicks outside the element in JavaScript, it is important to understand how JavaScript handles events.
When you click anywhere on a webpage, the event is first triggered for the target element where you can click, then it bubbles up to its parent element. This is known as the event bubbling in JavaScript. At the same time, event capturing also happens, where the event goes from the top-most parent to the target element. You can use event bubbling to detect when a user clicks outside an element.
Detecting Click Outside Element in JavaScript
JavaScript is the best for web development. It allows you to detect clicks that happen outside the element using the document.addEventListener(), and this is the simplest way to detect clicks outside an element.
Example 1: Closing the dropdown when clicking outside.
Output:

Explanation: In this example, clicking a button calls the dropdown menu (.dropdown-menu). And clicking anywhere outside the menu closes it. The event.stopPropagation() stops the click event from moving to the document. In other words, it is used to stop event bubbling.
Example 2: Closing a modal when clicking outside.
Output:
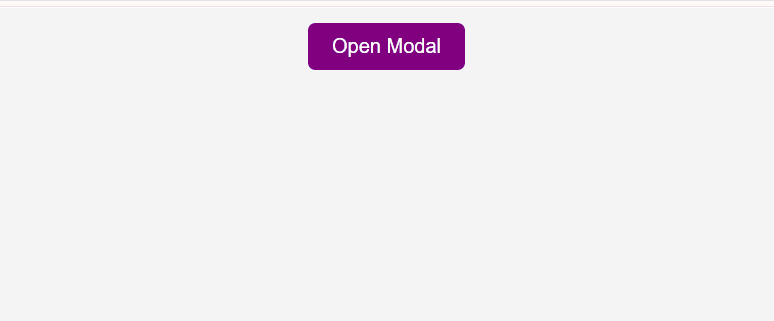
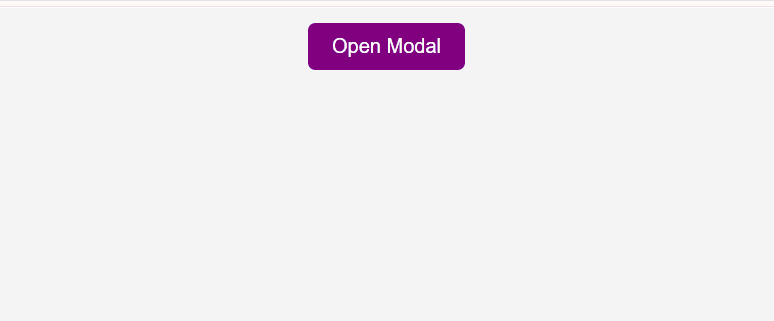
Explanation: In this example, you are creating a modal with the use of the eventListeners, event.target, and the contains() method.
Best Practices to Detect Click Outside Element
To get a good performance and a better user experience of the project, these are some best practices that you need to follow:
- Use stopPropagation() When Needed
- Instead of adding multiple event listeners, add a single event listener on the document and handle multiple elements inside it
Conclusion
Detecting clicks outside the HTML element is important for making various UI components like dropdowns, modals, and tooltips. JavaScript provides you a method to do this by using the document.addEventListener() and event.target.contains(). And by understanding these methods and learning best practices, you are able to detect clicks outside an element efficiently.
Detect Click Outside Element in JavaScript – FAQs
Q1. How do I detect clicks outside the multiple elements?
To detect clicks outside multiple elements, you can check whether the clicked element is inside any of the target elements using the some() and contains() methods.
Q2. How do I prevent event listeners from running multiple times?
To prevent event listeners from running multiple times, ensure that you remove event listeners when they are no longer needed. and avoid adding the same listener continuously inside a function that runs multiple times.
Q3. Can I detect clicks outside the element without using JavaScript?
No, using HTML or CSS only, you cannot detect clicks outside an element.
Q4. How do you show that an element is clickable?
To show the element is clickable, you can use CSS properties like the cursor: pointer or the :hover class.
Q5. What is the difference between click and onclick?
The onClick is a DOM property that triggers a function when the button is clicked, while click is an event name used with addEventListener().