Answer: We can remove a key from a dictionary by using del() and pop() functions.
It is important to remove a key from a Python dictionary because in certain circumstances some unnecessary key value has to be removed based on conditions. So, these methods along with some other techniques can remove keys from Python efficiently.
Table of Contents:
Methods to Remove a Key from a Python Dictionary
Python provides various built-in methods to remove a key from a dictionary. Following are some of these methods explained with examples:
Method 1: Using del( ) method to Remove a Key from a Dictionary in Python
The del( ) method will help you remove the key value directly from the dictionary. This will make sure the value does not return to the dictionary again which makes the data perfect and compatible.
Example:
Output:
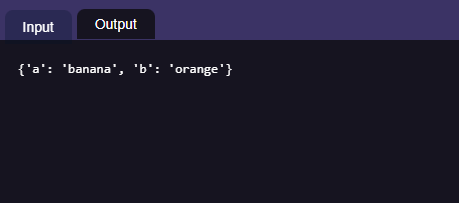
In this output you can see that value ‘c’ has been deleted and other than c everything has been displayed.
Method 2: Using pop( ) method to Remove a Key from a Dictionary in Python
The pop( ) method in Python will pop the key from the dictionary but will retain the value associated with the key. Unlike the del( ) method, this will allow the user to use the key elsewhere.
Example:
Output:
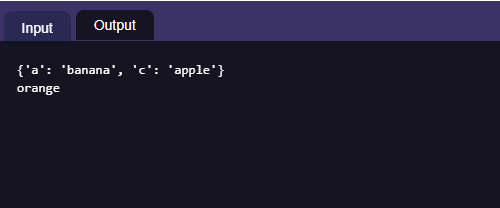
In this output, we can see that the key has been popped out but the value that the key contained has been retained and displayed.
1. Using dict.pop( ) to Remove a Key from a Dictionary in Python
The dict.pop( ) in Python, will remove the key value from the dictionary. It will remove the desired value. But if the value is not found it will state as a key value error.
Example:
Output:
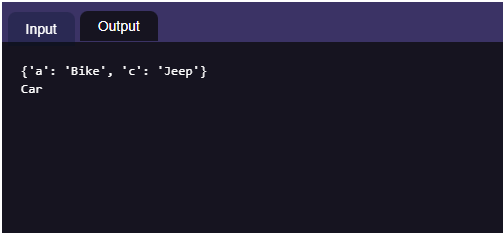
Let’s see if the value is not found in the dictionary,
Example:
Output:
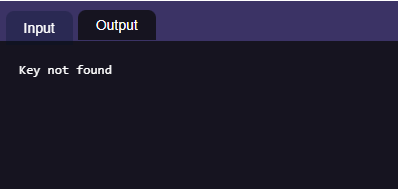
If the desired key value is not found in the dictionary it will execute as key value not found.
2. Using popitem( ) to Remove a Key from a Dictionary in Python
The popitem( ) in Python will help you remove the most recently added item from the dictionary. It uses the LIFO- Last In, First Out method to remove the last element in the dictionary.
Example:
Output:
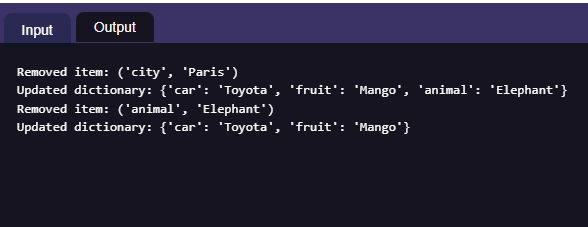
Method 3: Using filter( ) with lambda function to Remove a Key from a Dictionary in Python
You can use the filter() function along with a lambda function in Python, to filter elements from a dictionary based on conditions. It only keeps the data if it is true else remove it from the dictionary.
Example:
Output:
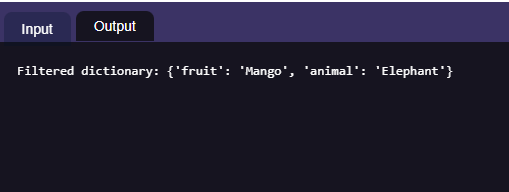
Method 4: Using Dictionary Comprehension to Remove a Key from a Dictionary in Python
We can remove the key from the dictionary in Python using dictionary comprehension, it will remove the key value we wanted and create a new key-value dictionary.
Example:
Output:
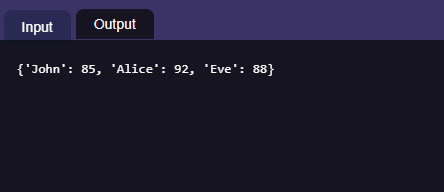
Method 5: Using clear( ) to Clear All the Keys from a Dictionary in Python
The clear( ) method in Python will clear all the dictionaries in the data using the keyword .clear(). This .clear() removes all key-value pairs from the dictionary.
Example:
Output:
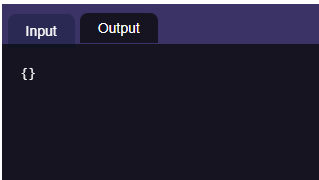
This cleared all the data that were present in the grades by using grades.clear( ). This is a straightforward method and is not advisable as it clears all the dictionaries which if something goes wrong will erase all the data in the dictionary.
Method 6: Using dict.keys() – {key} for Immutable Key Removal in Python
This method creates a new dictionary excluding specific keys.
Example:
Output:
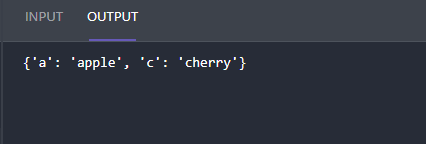
Method 7: Using copy() to Modify the Dictionary without Mutating the Original in Python
.copy() method can be used for creating a shallow copy of the dictionary.
Example:
Output:
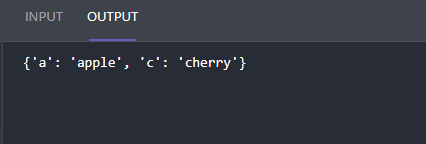
Conclusion
In conclusion, the most efficient ways to manage a dictionary in Python include using del() which is direct and efficient but raises KeyError if the key is missing, and pop() which removes the key but allows retrieving the value. The popitem() removes the most recently added key-value pair and dictionary comprehension is useful for non-destructive filtering. These tools, along with methods like filtering and dictionary comprehension, offer flexibility in utilizing and cleaning up dictionary data.