Using the Sequential model represents an easy approach to construct neural networks when working with deep learning models through Keras. Many layers can be arranged sequentially through this method with minimum complexity. Any neural network depends on an input layer to decide how data enters the system.
In this blog, we will teach you about the Keras Sequential model input layer, its importance, the different ways in which it can be defined, and its implementation. So, let’s get started!
Table of Contents
What is the Input Layer in the Keras Sequential Model?
Keras uses the input layer to establish data structure when developing neural network accession pipelines. Before reaching the rest of the model format conversion procedures, start with this initial layer.
Some Key Points about the Input Layer:
- The input layer serves as an entry point and does not process data.
- It is used to define the shape(dimensions) of the input data.
- It is created automatically during the time of specifying the shape of the first hidden layer. It can also be defined using Input().
Different Ways to Define the Input Layer
When implementing Keras Sequential models, users have two methods to define the input layer structure. They are explained below.
1. Implicit Input Layer (Defined in the First Layer)
When the shape of an input is specified within the initial hidden layer in Keras Sequential API it automatically generates an input layer.
Example:
Output:
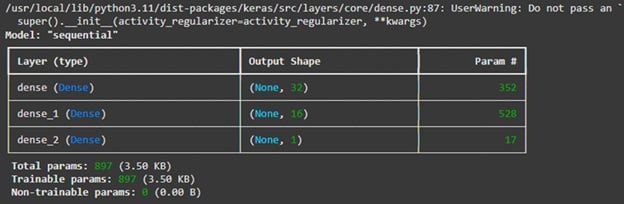
Explanation:
- The first Dense layer contains an input shape of the type (10,).
- During this operation, Keras generates an input layer following the specified shape (10,).
2. Explicit Input Layer using Input()
The Keras framework provides Input() as a function that enables you to specify the input layer. Additional input data control becomes possible with this data processing method.
Example:
Output:
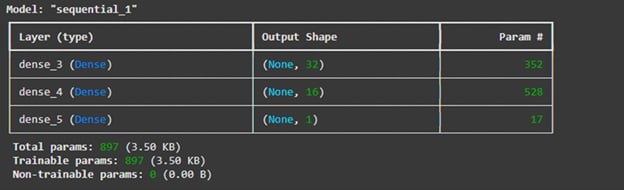
Explanation:
- The statement Input(shape=(10,)) specifies an input layer structure with dimensions of 10.
- Every other section of code remains equivalent to the input technique.
Choosing Between Implicit and Explicit Input Layers
Establishing the input layer stands vital in the Keras Sequential Model development processes. The precise model-data processing remains possible through this step. Keras offers two principal methods to define the input layer which include implicit and explicit definitions.
- An Implicit layer enables the use of the input shape when placed in the first hidden layer.
- The Explicit Layer can be utilized by defining the input layer independently through the Input() build function.
Keras Sequential Models provide two different approaches for defining the input layer which serve unique purposes in respective applications. The following discussion explains the correct use of each approach for defining an input layer together with its distinctive features.
1. Implicit Input Layer
You can use the Implicit Input Layer:
- Building a Simple Sequential Model remains your current task.
- You need no independent input layer in this scenario.
- The process of creating straightforward feedforward networks occurs at this time.
- You need to use this approach to create a streamlined model description.
Working of Implicit Input Layer:
The first hidden layer requires an input_shape argument to define its shape during the input layer configuration. The input shape parameter creates an input layer automatically in Keras according to this specified shape.
Example:
Output:
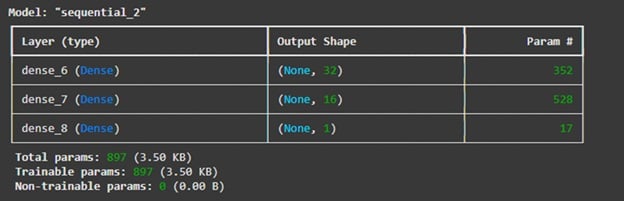
Explanation:
The above code is used to define a Keras Sequential model with an implicit input layer. It consists of three dense layers (32, 16, and 1 neurons, respectively). It uses ReLU and Sigmoid activations, and an input shape of (10,). Then it prints the summary of the model.
Advantages of Using Implicit Input Layer:
- They have simpler and shorter code: You don’t need to define a separate input layer.
- It is easier to read and understand basic models.
- It has less boilerplate code, hence it reduces the need for additional functional calls.
Disadvantages of Using Implicit Input Layer:
- It has less flexibility, hence it is unable to modify the input separately from the hidden layers.
- It is not suitable for complex architectures. It is harder to work with multiple inputs or custom data pipelines.
2. Explicit Input Layer using Input()
You can use the explicit input layer:
- While you are working with complex architectures, which require more flexibility.
- Your model utilizes multiple outputs when dealing with neural networks with multiple inputs or multimodal models.
- The process of utilizing Keras Functional API alongside its advanced features occurs during this time.
- The main reason to use this interface is to gain superior control of input layer processing.
Working of Explicit input layer:
In this method, you have to explicitly define an Input() layer at the beginning. This will help you to get better flexibility and clarity during the construction of more advanced models.
Example:
Output:
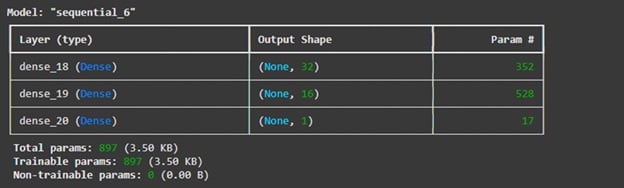
Explanation:
The above code is used to define a Keras Sequential model which has an explicit input layer ( Input(shape=(10,)) ). It is then followed by three Dense layers (32, 16, and 1 neurons, respectively) by using ReLU and Sigmoid activation functions. It prints the summary of the model at last.
Advantages of Using the Explicit Input Layer:
- It is more flexible and can modify the input separately from the hidden layers.
- It works well with multiple inputs, hence, it is suitable for complex models.
- It provides better compatibility with the functional API, which makes the integration easier.
- It allows preprocessing of data before feeding input into the model.
Disadvantages of Using Explicit Input Layers:
- It has a slightly longer code, which requires an additional definition for the layer.
- It is unnecessary for simple models, hence, it adds complexity where it may not be required.
Comparison between Implicit and Explicit Input Layer
Given below is a comparison between Implicit and Explicit Input layers in tabular form.
Implicit Input Layer | Explicit Input Layer |
Here, the input shape is defined in the first layer. | Here, the input shape is defined separately using Input(). |
It is suitable for simple, straightforward models. | It is suitable for complex architectures and multi-input models. |
The code is easier and shorter. | The code is slightly more complex. |
It is less flexible. | It is more flexible. |
It does not support multiple inputs. | It supports multiple inputs. |
Preprocessing is not possible before the first layer. | Preprocessing is possible before passing the data to the model. |
It has limited Functional API support. | It is fully compatible with functional API. |
Training a Keras Sequential Model with Input Layer
Now, let’s build and train a neural network using the Keras Sequential Model with an input layer.
Example:
Output:
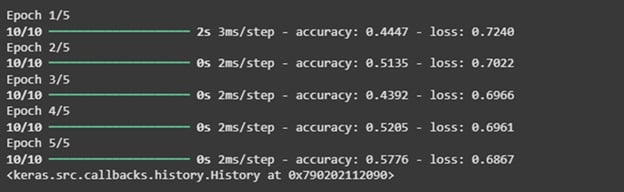
Explanation:
- Here, random training data is generated with 10 features.
- This model consists of an input layer with 10 features and 2 hidden layers.
- Then, the model is compiled using Adam Optimizer and trained for 5 epochs.
- The accuracy of the model gradually improves during training.
Conclusion
The Keras Sequential model input layer is an important component in defining the entering of data into a neural network. In this blog, we have explored two primary ways to define an input layer: the Implicit Input layer, where the first layer is hidden and the input shape is specified, and the Explicit input layer, where a separate input layer is used for more control. The Implicit approach is simple and more suitable for simple models, whereas the Explicit model provides greater flexibility, which also supports complex architectures, multi-input models, and preprocessing steps. For building a simple, sequential neural network, using an implicit layer is more efficient. Whereas, for more flexibility, using an explicit input layer with Input() is a better option. Therefore, having a better understanding of these differences will help you to design neural networks more efficiently and effectively in Keras.
FAQs
1. What is the role of the input layer in a Keras Sequential model?
The role of the input layer is to define the shape and structure of the data that enters the neural network. It helps to ensure that the model receives input in the correct format before it is processed through the hidden layers.
2. What is the difference between an implicit and an explicit input layer in Keras?
An implicit input layer is created automatically when the input_shape parameter is specified in the first layer.
An explicit input layer is defined separately by using Input(). This provides more flexibility for complex models.
3. When should I use an explicit input layer in a Keras model?
You can use an explicit layer while working with complex architectures and multiple inputs or when integrating with the Functional API.
4. Can I preprocess data using an implicit input layer?
No, you cannot. Implicit input layers do not allow preprocessing before the data is passed into the model. If there is any need for preprocessing, you can use an explicit input layer for greater control.
5. How do I specify the input shape in a Keras Sequential model?
You can specify the input shape either inside the first hidden layer by using input_shape=(features,) or you can do it explicitly by using Input(shape=(features,)) as the first layer in the Sequential model.